Introduction to Roblox Lua Scripting is a fundamental aspect of creating engaging and interactive experiences within the Roblox platform. As a game development environment, Roblox utilizes Lua, a lightweight and versatile programming language, to empower creators with the tools necessary to bring their visions to life. This tutorial aims to guide beginners through the process of understanding and utilizing Lua scripts in Roblox, providing a comprehensive foundation for further learning and development.
Understanding Lua Basics

Lua, short for “moon” in Portuguese, is designed to be simple, efficient, and easy to learn. Its syntax is straightforward, making it an ideal choice for beginners and experienced developers alike. In the context of Roblox, Lua is used to create scripts that can interact with the game environment, manipulate objects, and control the behavior of characters and other entities. To start scripting in Lua, one must first understand basic concepts such as variables, data types, loops, conditional statements, and functions.
Variables and Data Types
In Lua, variables are used to store and manipulate data. Unlike some other programming languages, Lua does not require explicit type definitions for variables. Instead, the data type of a variable is determined by the type of value assigned to it. Common data types in Lua include numbers, strings, booleans, tables, and functions. For example, to declare a variable named “playerName” and assign it a string value, you would use the following syntax: local playerName = “JohnDoe”. This illustrates how variables can be used to store and later reference specific pieces of information within a script.
Data Type | Description | Example |
---|---|---|
Number | Numeric values | local age = 25 |
String | Text values | local greeting = "Hello, World!" |
Boolean | True or False values | local isAdmin = true |
Table | Collection of key-value pairs | local playerData = {name = "Jane", score = 100} |
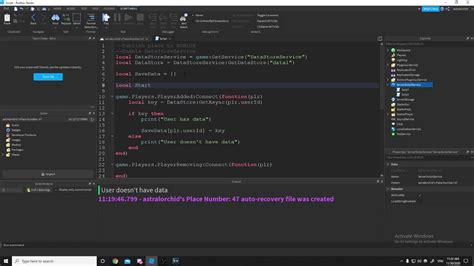
Scripting in Roblox

Roblox provides several types of scripts, each serving a different purpose. LocalScripts run on the client side, meaning they execute on the player’s device, which is useful for creating user interfaces or handling local events. Script, on the other hand, runs on the server side, allowing for the manipulation of the game world and synchronization of data across all clients. ModuleScripts are reusable pieces of code that can be required by other scripts, promoting modularity and code reuse.
Creating a Simple Script
To demonstrate the basic principles of scripting in Roblox, consider a simple example where a part (a 3D object) changes color when a player touches it. This can be achieved by attaching a Script to the part and using the Touched event to detect when a character comes into contact with it. The script would listen for the Touched event, check if the object that touched the part is a character, and then change the part’s color accordingly.
Here's an example of how such a script might look:
-- Get the part that this script is attached to
local part = script.Parent
-- Function to change the part's color
local function changeColor()
part.BrickColor = BrickColor.new("Red")
end
-- Listen for the Touched event
part.Touched:Connect(function(hit)
-- Check if the object that touched the part is a character
if hit.Parent:FindFirstChild("Humanoid") then
changeColor()
end
end)
Key Points
- Understanding Lua basics is essential for scripting in Roblox.
- Variables and data types are fundamental concepts in programming.
- Roblox scripts can run on the client or server side, each with its use cases.
- Creating simple interactions, like changing a part's color, introduces developers to event-driven programming.
- Modules and script organization are crucial for large-scale projects.
Advanced Scripting Concepts
As developers become more comfortable with the basics, they can delve into more advanced topics such as object-oriented programming, networking, and data storage. Roblox’s API provides a wide range of functionalities that can be leveraged to create complex and engaging game mechanics. For instance, using RemoteEvents and RemoteFunctions allows for secure communication between the client and server, enabling features like real-time multiplayer interactions and server-side validation of client actions.
Object-Oriented Programming
Object-oriented programming (OOP) is a paradigm that organizes software design around objects and the interactions between them. In Lua, OOP can be implemented using tables and metatables, which allow for the creation of classes and objects. This programming style is particularly useful in Roblox for managing complex game logic and creating reusable code modules.
What is the primary purpose of using scripts in Roblox?
+The primary purpose of using scripts in Roblox is to create interactive and dynamic game elements, such as character movements, object manipulations, and user interface interactions.
How do I get started with scripting in Roblox?
+To get started with scripting in Roblox, begin by learning the basics of Lua, then experiment with simple scripts within the Roblox Studio environment. The official Roblox documentation and community tutorials are valuable resources.
What are some advanced topics in Roblox scripting?
+Advanced topics in Roblox scripting include object-oriented programming, networking with RemoteEvents and RemoteFunctions, and data storage using DataStoreService. Mastering these topics can significantly enhance the complexity and engagement of your games.
In conclusion, scripting in Roblox with Lua is a powerful tool for game development, offering a wide range of possibilities from simple interactions to complex, multiplayer experiences. By mastering the basics of Lua, understanding the different types of scripts in Roblox, and exploring advanced topics, developers can unlock their full creative potential and bring unique, engaging experiences to the Roblox community.